This article will address a common question around Python – Delete File. When working with files in Python, there is often a requirement to be able to delete them – the files may be temporary and need to be cleaned up after the program has finished, or it may be that the python program is designed to search for and delete files in a particular location. In this article I will run through some examples of how you can delete files using Python.
Deleting Files using the Python os.remove function
The preferred way to delete a file is to use the os.remove function. First of all I will create a dummy file that we can use to test with:
touch filetodelete.txt
Now in my python session I need to import the os module then run the os.remove function to delete the file:
import os
os.remove("filetodelete.txt")
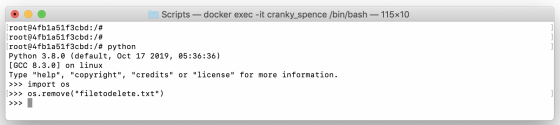
Simple as that! The file is gone! But, this method is a little gung-ho. It works great if you know for sure the file you wish to delete exists (for example you may have just created it earlier in your python code), but what if you’re not sure if it is present? Then you can build a check into your code, to check that the file exists before deleting it.
Confirming the File Exists before Deleting it with Python
We can use the os.path.exists function to do this:
import os
if os.path.exists("filetodelete.txt"):
os.remove("filetodelete.txt")
Using the IF statement we can confirm the file exists before deleting it. You could then add an ELSE statement to this to get your python code to perform a different action if the file doesn’t exist.
Delete a Directory Using Python
You can also use Python to delete a directory. Again, using the os module, we can use the os.rmdir function:
import os
os.rmdir("directorytodelete")
But, be aware that you can only delete directories that do not contain any files using this method.
Delete all Files in a Directory using Python
To delete all files in a given directory, we can use the python glob module along with the os module. The python glob module allows us to match files based on a unix expression. You can read more about glob here. We can use this pattern matching functionality to match all files in a given directory then use the os.remove function (in a loop) to remove each one. For example:
import os
import glob
files = glob.glob('/YOUR/PATH/*')
for f in files:
os.remove(f)
The ‘/YOUR/PATH/*’ in the code will specify all files in your given path. NOTE: use caution with this, ensure you test this before using in a production setting!! Always use caution when automating file deletes!
Deleting Files with a Given Extension
Using the same method as described above you an delete files with a particular file extension – we just need to change the pattern match in the code. For example, to delete all ‘gif’ files we can use:
import os
import glob
files = glob.glob('/YOUR/PATH/*.gif')
for f in files:
os.remove(f)
This should delete all files with a .gif extension, whilst leaving other files in place.As above, use caution with this, ensure you test this before using in a production setting to ensure it works as you expect!!
Thanks for reading! Hopefully this has given you some tips on how to delete files and directories using python! Happy coding! If you want some tips on adding comments to your code, check out this article.