NSX-T Manager provides a REST API, using JSON object encoding , which can be used to automate management activities. Clients can interact with the API using RESTful web service calls over the HTTPS protocol. This article will take a look at how the PowerShell Invoke-RestMethod
cmdlet can be used to make API requests to NSX-T Manager to retrieve information about the NSX-T environment, and how we can use it in a working PowerShell script.
Note: the examples used here were tested in an environment using PowerShell 6 and NSX-T 3.1.
How to Authenticate the PowerShell API Connection
In order to be able to make REST API requests we will need to be able to authenticate to NSX Manager. To do so, I will set some variables to contain the username and password needed to connect. For the username, I am using the built in admin account (though in production you should use an account with only enough permissions to do what you need), and we are capturing the value for the password interactively.
$username = "admin"
$pass = Read-Host "Enter password" -AsSecureString
Next, we need to take the inputted password value and write it to a variable we can use, whilst converting the SecureString
to a string using the SecureStringToBSTR
method.
$password = [System.Runtime.InteropServices.Marshal]::PtrToStringAuto([System.Runtime.InteropServices.Marshal]::SecureStringToBSTR($securedValue))
Now we can use this to build our username:password
which we will use later:
$userpass = $username + ":" + $password
Next we can move on to building the header we will be sending in the API request.
Building the Request Header
Now we need to craft the header to send when making the API request. This header needs to include the credentials needed to authenticate to NSX manager, but for it to work we need to encode the contents of our $userpass
variable (the username and password pair) into Base64. We can use this using the following:
$bytes= [System.Text.Encoding]::UTF8.GetBytes($userpass)
$encodedlogin=[Convert]::ToBase64String($bytes)
$authheader = "Basic " + $encodedlogin
$header = New-Object "System.Collections.Generic.Dictionary[[String],[String]]"
$header.Add("Authorization",$authheader)
If we check the contents of the $header
variable at this stage we should see something like:
$headers
Key Value
--- -----
Authorization Basic YWRtaW46Nrv3d1N2YUghMnVMTm5QcVZlbQ==
Looks good! Now with the request header crafted, we can finally use invoke-restmethod to make our request.
Using Invoke-RestMethod with NSX-T API
Before we make the request, we need the URI to which to send our request. In this example I am going to use https://nsxmanager/api/v1/logical-routers
(replace nsxmanager with your manager IP/hostname!). This URI will can be used to work with the logical routers in the NSX-T environment. Check out the NSX-T REST API explorer to find out what URI to use for just about any NSX-T resource/function.
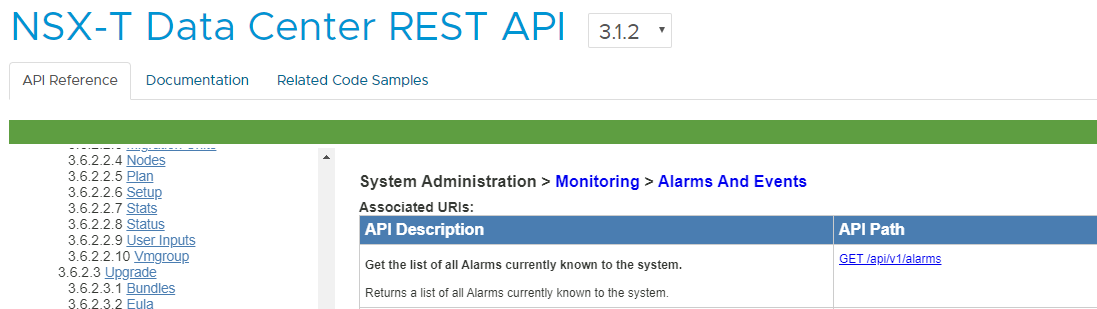
Ok, so lets set up a variable containing the URI we wish to work with:
$uri = "https://192.168.0.10/api/v1/logical-routers"
Now we can make our request using invoke-restmethod
, and storing the output in a variable ($res
):
$res = Invoke-RestMethod -Uri $uri -Headers $header -Method 'GET'
Now, if we display the contents of the $res variable (with a SELECT statement, to filter the information), we can see the result of the REST API query:
$res.results | select display_name, router_type, high_availability_mode
display_name router_type high_availability_mode
------------ ----------- ----------------------
T0 TIER0 ACTIVE_STANDBY
We have successfully retrieved a list of the logical routers configured in this NSX-T deployment!
Putting it all together
So, what does the complete script look like? Here we go:
$username = "admin"
$pass = Read-Host "Enter password" -AsSecureString
$password = [System.Runtime.InteropServices.Marshal]::PtrToStringAuto([System.Runtime.InteropServices.Marshal]::SecureStringToBSTR($pass))
$userpass = $username + ":" + $password
$bytes= [System.Text.Encoding]::UTF8.GetBytes($userpass)
$encodedlogin=[Convert]::ToBase64String($bytes)
$authheader = "Basic " + $encodedlogin
$header = New-Object "System.Collections.Generic.Dictionary[[String],[String]]"
$header.Add("Authorization",$authheader)
$uri = "https://192.168.0.10/api/v1/logical-routers"
$res = Invoke-RestMethod -Uri $uri -Headers $header -Method 'GET'
$res.results | select display_name, router_type, high_availability_mode
Hopefully this has given you an idea of how you can interact with the NSX-T REST API using PowerShell!